Overview
While native iOS keyboards have few ways to customize the look and feel, KeyboardKit keyboards can be styled and customized to great extent.
KeyboardKit uses KeyboardStyle
types to style its views. For instance, a KeyboardStyle.InputCallout
style can be used to style a Callouts.InputCallout
view.
KeyboardKit Pro unlocks a powerful theme engine, and many more ways to style a keyboard.
Keyboard Style Namespace
KeyboardKit has a KeyboardStyle
namespace that contains style-related types. It will probably be removed in KeyboardKit 9, together with the KeyboardStyleService
, and replaced by view modifiers.
Keyboard Style Services
A KeyboardStyleService
can provide dynamic styles for different parts of a keyboard. Unlike static styles, a style service can vary styles depending on KeyboardContext
, KeyboardAction
, etc.
Color & Image Extensions
KeyboardKit defines additional, keyboard-specific Color
and Image
extensions, etc. to make it easy to create keyboards that look like a native iOS keyboard.
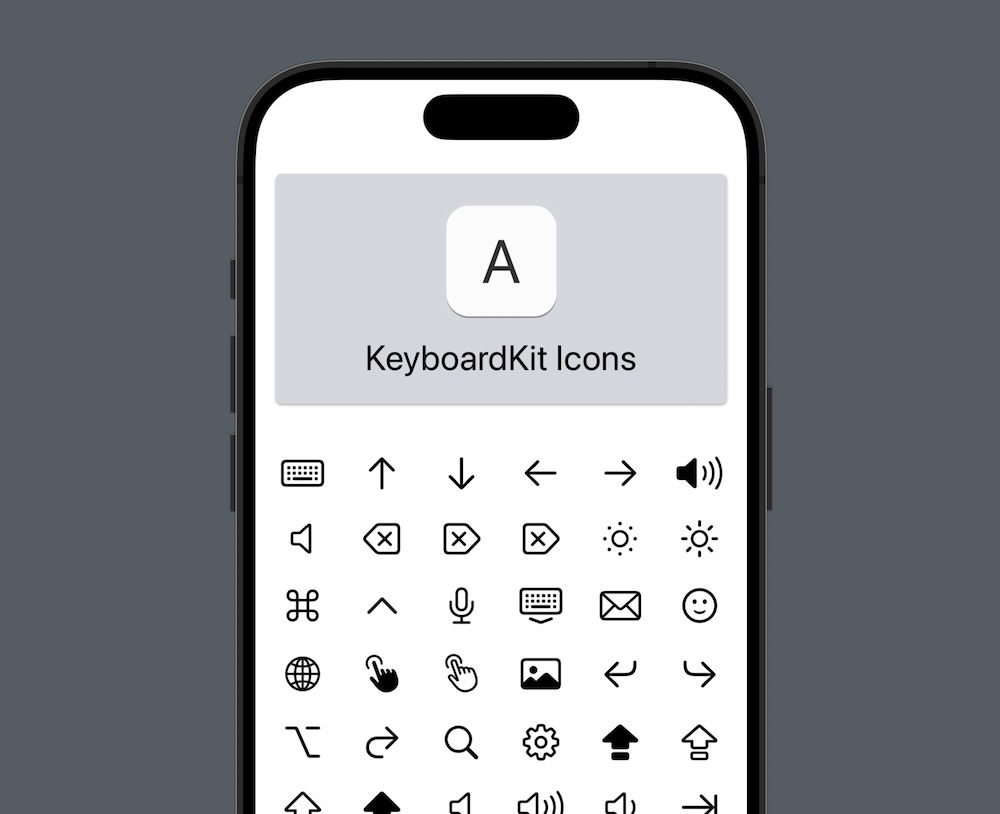
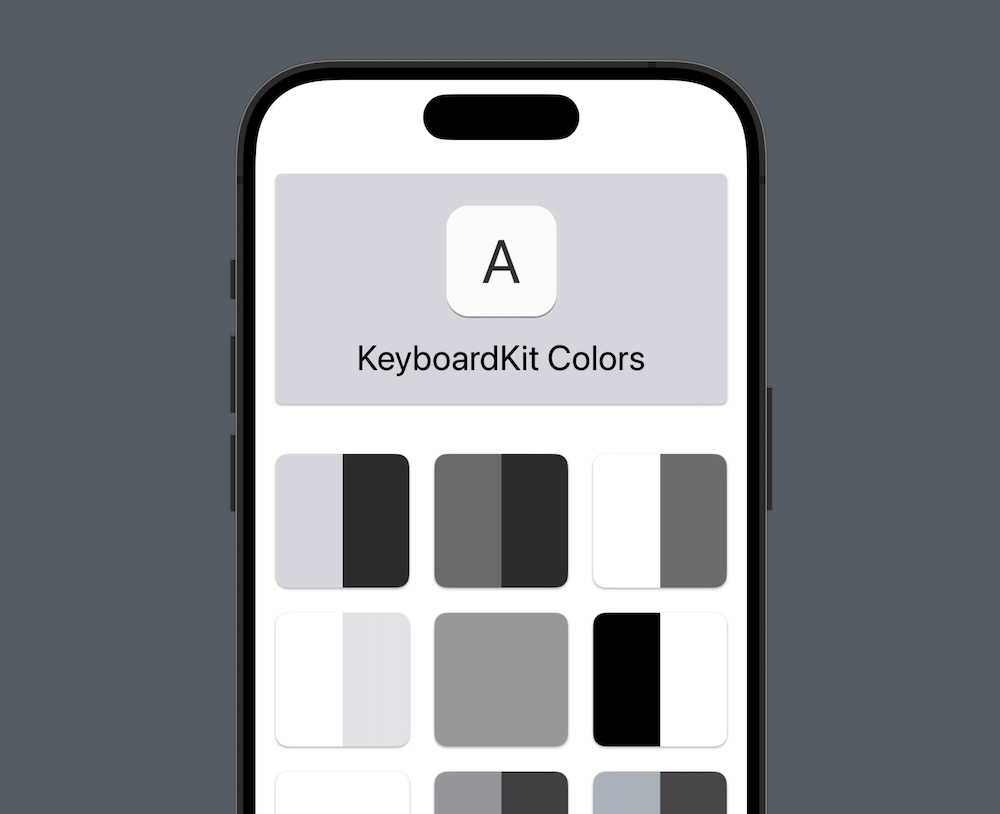
KeyboardKit defines contextual colors that take a KeyboardContext
, like keyboardBackground(for:)
, that vary the color result based on the context. Prefer context-based colors whenever possible.
See the documentation for more information about colors and images, including some important information about how some colors are semi-transparent to work around a system bug in iOS.
Type Extensions
Many KeyboardKit types define standard images, texts & colors. For instance you can get a standard image and text for a KeyboardAction
with standardButtonImage(for:)
and standardButtonText(for:)
:
let context = KeyboardContext()
let image = KeyboardAction.command.standardButtonImage(for: context) // Command icon
let text = KeyboardAction.space.standardButtonText(for: context) // KKL10n.space
View styles
KeyboardKit defines custom styles for its various view. For instance, the Keyboard
Keyboard/Button
view has a Keyboard/ButtonStyle
that can be applied with the SwiftUICore/View/keyboardButtonStyle(_:)
view modifier.
Most views have static, standard styles that can be replaced by custom styles to change the global default style for that particular view.
π KeyboardKit Pro
KeyboardKit Pro unlocks additional image assets, and a KeyboardTheme
engine that makes easier to style keyboards with themes.
Emoji Icons
KeyboardKit Pro unlocks vectorized emoji assets for all EmojiCategory
s, for instance keyboardEmoji
& emojiCategory(_:)
:
Since these images are vectorized, they scale well when they are resized. They however do not support different font weights, so do not render them alongside other SF Symbols.
Themes
KeyboardKit Pro unlocks a KeyboardTheme
engine that makes easier to style keyboards with themes:
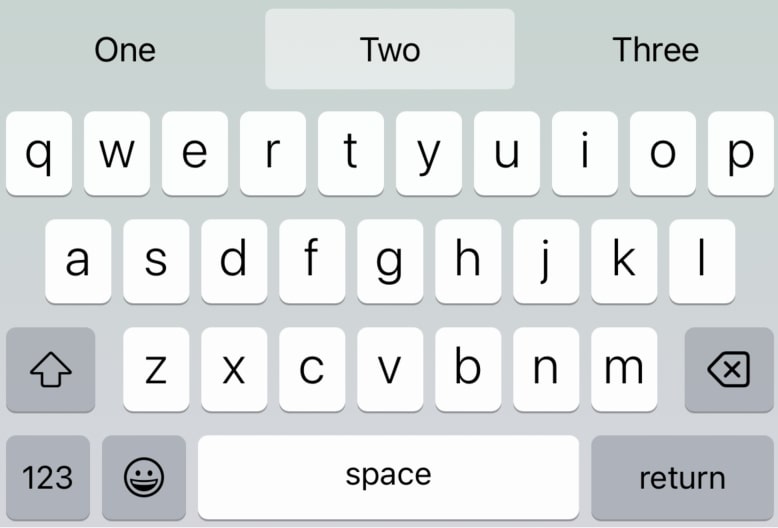
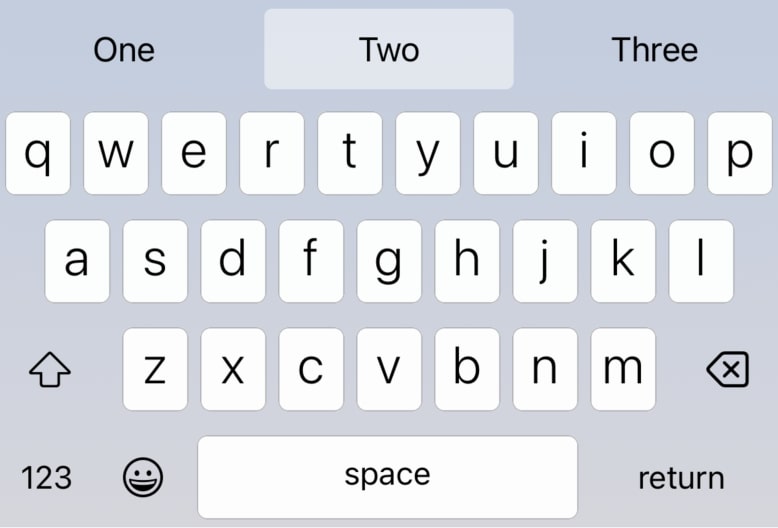
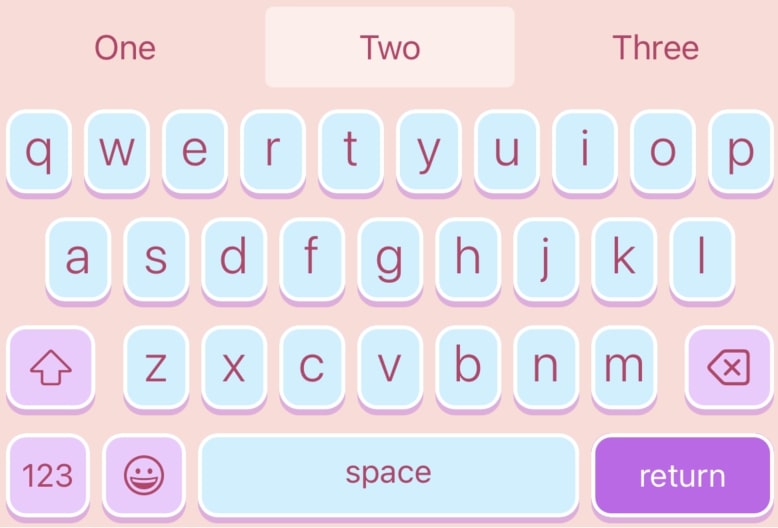
See themes for more information.
Documentation
The information on this page is shortened to be easier to overview. For more information see the online documentation.
Core Features
KeyboardKit is packed with features to help you build amazing custom keyboards. You can upgrade to KeyboardKit Pro to unlock pro features.
Essentials
KeyboardKit provides essential features, utilities & views.
Pro Features
KeyboardKit Pro unlocks features like autocomplete, emojis, AI, etc.
Actions
KeyboardKit makes it easy to handle keyboard actions.
App
KeyboardKit makes it easy to set up your app and keyboard.
Callouts
KeyboardKit can show input & secondary action callouts.
Device Utilities
KeyboardKit has device-specific utilities.
Emojis
KeyboardKit defines emojis, emoji categories, skin tones, etc.
Feedback
KeyboardKit can trigger audio & haptic feedback.
Gestures
KeyboardKit has a customizable keyboard gesture engine.
Host
KeyboardKit can identify the host application.
Layout
KeyboardKit has customizable input sets & keyboard layouts.
Localization
KeyboardKit supports 60+ locales and languages.
Navigation
KeyboardKit lets you open urls and apps from the keyboard.
Previews
KeyboardKit has extension keyboard preview support.
Proxy Utilities
KeyboardKit makes your text document proxy do a LOT more.
Settings
KeyboardKit has tools for in-app settings & System Settings.
Status
KeyboardKit detects if a keyboard is enabled, has full access, etc.
Styling
KeyboardKit lets you style your keyboards to great extent.
π Pro Features
KeyboardKit Pro unlocks pro features that take your keyboard to the next level. Go pro today!
Essentials
KeyboardKit Pro unlocks system keyboard and theme previews.
AI Support
KeyboardKit Pro unlocks features that are needed for AI.
App
KeyboardKit Pro unlocks app-specific screens & views.
Autocomplete
KeyboardKit Pro unlocks on-device & remote autocomplete.
Callouts
KeyboardKit Pro unlocks localized callouts for all locales.
Dictation
KeyboardKit Pro can trigger dictation from the keyboard.
Emoji Keyboard
KeyboardKit Pro unlocks an emoji keyboard.
External Keyboards
KeyboardKit Pro can detect if an external keyboard is being used.
Host
KeyboardKit Pro can identify and open specific host applications.
Layout
KeyboardKit Pro unlocks localized layouts for all locales.
Localization
KeyboardKit Pro unlocks support for 60+ locales.
Previews
KeyboardKit Pro unlocks system keyboard and theme previews.
Proxy Utilities
KeyboardKit Pro makes the proxy able to read the full document.
Text Input
KeyboardKit Pro unlocks tools to let you type within the keyboard
Themes
KeyboardKit Pro unlocks a theme engine & many standard themes.